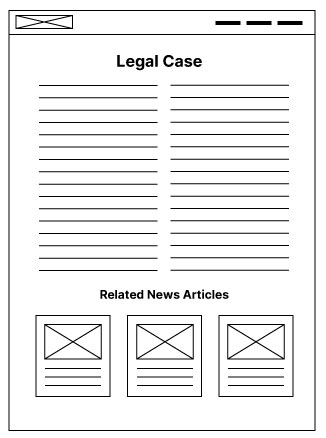
Let’s say you have a website with legal cases and you want to credit specific news articles at the bottom of each case page. Both the news articles and the legal cases use custom post types. So you just want to be able to select the associated news articles when you’re creating a case post.
How do you do this with Elementor and Advanced Custom Fields (ACF)?
While it’s easy to set up a post loop in Elementor and query one of your custom post types, you’d have to know exactly which posts you’d like to query or choose to query all of them in a specific order. Which doesn’t work well when you’re using it on a post page template, since each page might have different posts you want to query.
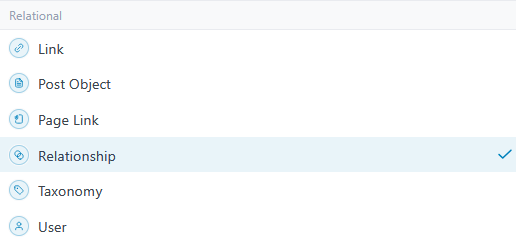
There’s currently no built in way to adjust that query based on an ACF output. Which is too bad, since ACF includes several different types of fields that relate posts together.
This doesn’t mean it’s impossible, just that we have to code it with our PHP functions instead.
To understand this particular dev solution you already need an understanding of Elementor loops, how to set up a loop grid on your page, and how to adjust the query settings. You’ll also need to already have an understanding of how to set up custom post types, custom taxonomies, and create fields for custom post types in ACF. And how to add code to the functions file of your website. If you’re not comfortable with changing the functions file you can also use a helpful plugin like Code Snippets to add custom PHP instead.
The Elementor Custom Query Filter
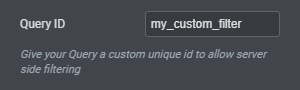
Fortunately Elementor does have a custom query filter built in to help us code this with some helpful examples of ways we might use it. This can allow you to add multiple post types into the same post query, to filter posts by meta data, and order by comment count as a few of the examples.
So the first step here is to add our custom query ID. In this case I’m using 'news_articles_attached'
as my ID since that’s what I’m doing.
function my_query_by_post_types( $query ) {
//replace news_article with your custom_post_type ID
$query->set( 'post_type', 'news_article' );
}
add_action( 'elementor/query/news_articles_attached', 'my_query_by_post_types' );
//replace news_articles_attached with your custom query ID
It’s a good idea to make sure your code is working first also good to try out one a simple version to make sure your hooks are all correct. This code means my loop will query all the news articles. The number of articles and order are determined by what I’ve already setup in my loop on the Elementor side.
Querying the Posts using a Custom Taxonomy
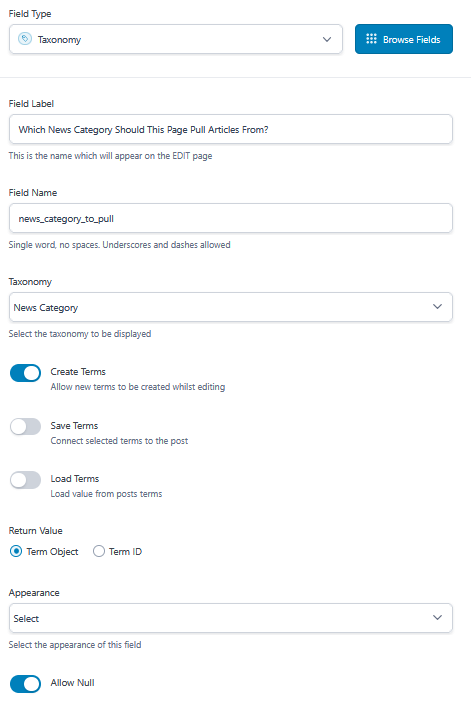
Next we need to add an ACF field to our Legal Case posts that can let us define the related news articles for each case. There are different fields to use depending on your needs, but in this case I went with the Taxonomy field, since the news articles are already categorized based on case through a custom taxonomy.
If you don’t yet have a custom taxonomy for the post type you’re querying you’ll need to create one through ACF for this to work.
I’m adding the Taxonomy field to my Legal Cases field group, so that it associates the News Article taxonomy with those pages.
I decided I wanted my client to be able to create new terms in that taxonomy for their convenience, but there’s no reason to have it connect those terms to my Legal Cases since they don’t use the news article taxonomy for filtering. And since I only want one term selected at a time the appearance is set to “Select”. I’m also allowing the cases pages to have no articles associated, and in that case “Allow Null” is okay for this project.

This creates an ACF field on my Cases pages to select which news articles they want to query.
The Code
Now we have all the pieces set up, and it’s just a matter of making sure out function queries our selected news category in our post loop.
do_action_ref_array( ‘pre_get_posts’, WP_Query $query )
We’re going to use an existing 'pre_get_post'
hook called 'tax_query'
to query our taxonomy. This means it’ll do this action before it gets the posts. The action we want to do is $query->set
. Where it gets a bit tricky is that 'tax_query'
is an array that requires taxonomy, field, and terms to be selected, so we need use a variable to set it, instead of it being more straightforward like 'post_type'
is $query->set( 'post_type', 'category_ID' );
.
In order to pull the ACF field we need to use another function that will allow us to pull the individual taxonomy term that was selected. Normally you would use 'terms' => 'category_term'
and just pick one of the ID’s from the taxonomy terms. But here we have to use an additional function to pull the ID get_field('ACF_field_name')
.
This makes our final code:
function my_query_by_post_types( $query ) {
$tax_query[] = [
array(
array(
//replace 'news-category' with the custom taxonomy's ID
'taxonomy' => 'news-category',
'field' => 'slug',
//replace 'news_category_to_pull' with the ACF category field ID
'terms' => get_field('news_category_to_pull'),
),
),
];
$query->set( 'tax_query', $tax_query );
}
//replace 'news_articles_attached' with your custom query ID
add_action( 'elementor/query/news_articles_attached', 'my_query_by_post_types' );
This should allow you to query the post loop on your custom post type’s page based off of a separate custom post type’s taxonomy.
Happy coding!